MDX Kitchen Sink
A collection of all the features of MDX
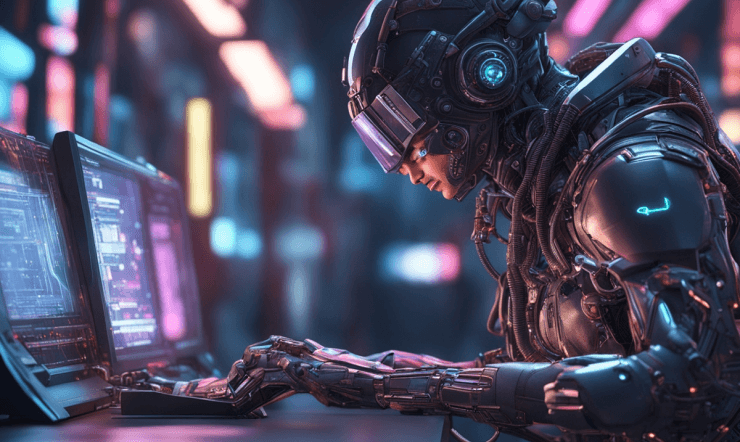
Written on
By 0xAquaWolf
Last updated
Table of Contents
rehype-pretty-code
is a Rehype plugin powered by the
shiki
syntax highlighter that provides beautiful code blocks for Markdown or MDX. It works on both the server at build-time (avoiding runtime syntax highlighting) and on the client for dynamic highlighting.
Editor-Grade Highlighting
Line Numbers and Line Highlighting
Draw attention to a particular line of code.
import { useFloating } from "@floating-ui/react";
function MyComponent() {
const { refs, floatingStyles } = useFloating();
const testFunction = () => {
return 'test';
}
return (
<>
<div ref={refs.setReference} />
<div ref={refs.setFloating} style={floatingStyles} />
</>
);
}
Word Highlighting
Draw attention to a particular word or series of characters.
import { useFloating } from "@floating-ui/react";
function MyComponent() {
const { refs, floatingStyles } = useFloating();
return (
<>
<div ref={refs.setReference} />
<div ref={refs.setFloating} style={floatingStyles} />
</>
);
}
ANSI Highlighting
vite v5.0.0 dev server running at:
> Local: http://localhost:3000/
> Network: use `--host` to expose
ready in 125ms.
8:38:02 PM [vite] hmr update /src/App.jsx
Inline ANSI: > Local: http://localhost:3000/
Kitchen Sink Meta Strings
isEven.js
function isEven(number) {
if (number === 1) {
return false;
} else if (number === 2) {
return true;
} else if (number === 3) {
return false;
} else if (number === 4) {
return true;
} else if (number === 5) {
return false;
} else if (number === 6) {
return true;
} else if (number === 7) {
return false;
} else if (number === 8) {
return true;
} else if (number === 9) {
return false;
} else if (number === 10) {
return true;
} else {
return "Number is not between 1 and 10.";
}
}
// Example usage:
console.log(isEven(3)); // Should return false
console.log(isEven(4)); // Should return true
console.log(isEven(11)); // Should return "Number is not between 1 and 10."
import { cn } from '@/lib/utils';
import { ReactNode } from 'react';
interface CalloutProps {
children?: ReactNode;
type?: 'default' | 'warning' | 'danger';
}
export function Callout({
children,
type = 'default',
...props
}: CalloutProps) {
return (
<div
className={cn(
'boder-l-4 my-6 w-full items-start rounded-md border p-4 dark:max-w-none',
{
'dark:prose border-red-900 bg-red-50': type === 'danger',
'dark:prose border-yellow-900 bg-yellow-50': type === 'warning',
},
)}
{...props}
>
<div>{children}</div>
</div>
);
}
Exmplae MyComponent
import { format, parseISO } from 'date-fns';
import Image from 'next/image';
import SVGGradientBg from '@/components/Hero/SVGGradientBg';
import Menu from '@/components/Hero/Menu/Menu';
import BlogViewsIcon from '@/public/images/svg/BlogViewsIcon.svg';
import ReadTimeIcon from '@/public/images/svg/ReadTimeIcon.svg';
import { notFound } from 'next/navigation';
import { getPostBySlug } from '@/lib/mdx';
import markdownToHtml from '@/lib/markdownToHTML';
const PostLayout = async ({ params }: { params: { slug: string } }) => {
const post = getPostBySlug(params.slug);
if (!post) notFound();
let content = '';
if (post) content = await markdownToHtml(post?.content);
return (
<div className="relative overflow-x-hidden">
<article className="mx-auto max-w-5xl py-8 text-white">
<SVGGradientBg />
<div className="mx-auto max-w-[1440px] px-4 sm:px-6 lg:px-8">
<Menu />
</div>
<div className="lg:mt-30 relative z-10 mb-8 mt-10 px-2 text-center">
<h1 className="inline-block bg-gradient-to-t from-[#F26FD8] to-[#FFF4F4] bg-clip-text text-2xl font-bold text-transparent lg:text-6xl">
{post.title}
</h1>
<p className="mb-10 mt-2 text-sm text-white lg:text-lg">
{post.description}
</p>
<div className="relative">
<Image
src={post.imageUrl}
alt={post.title}
width={1000}
height={1000}
className="rounded-2xl"
/>
<div className="absolute bottom-0 left-0 right-0 top-0 rounded-2xl bg-black/50" />
<div className="absolute bottom-3 left-3 mt-4 flex justify-center space-x-4 text-xs text-white lg:bottom-10 lg:left-10">
<div className="flex flex-col">
<div className="flex items-center space-x-2 text-sm lg:text-xl">
<div className="flex items-center space-x-2">
<Image
src={ReadTimeIcon}
alt="Read Time"
width={16}
height={16}
/>
<span className="bg-gradient-to-r from-[#FCD3ED] to-[#F690DF] bg-clip-text font-medium text-transparent">
{post.readTime} min read
</span>
</div>
<div className="flex items-center space-x-2">
<Image
src={BlogViewsIcon}
alt="Blog Views"
width={16}
height={16}
/>
<span className="bg-gradient-to-r from-[#FCD3ED] to-[#F690DF] bg-clip-text font-medium text-transparent">
53,000 views
</span>
</div>
</div>
<div className="mt-2 flex items-center justify-center space-x-1 text-sm lg:text-xl">
<div className="m-0">Written on</div>
<time dateTime={post.date} className="m-0">
{format(parseISO(post.date), 'LLLL d, yyyy')}
</time>
<div className="m-0">By 0xAquaWolf</div>
</div>
<div className="mt-2 flex items-center space-x-1 text-xs lg:text-lg">
<div className="m-0">Last updated</div>
<time dateTime={'2024-09-12T00:00:00.000Z'} className="m-0">
{format(
parseISO('2024-09-12T00:00:00.000Z'),
'LLLL d, yyyy',
)}
</time>
</div>
</div>
</div>
</div>
</div>
<div className="z-99 prose-md prose prose-invert relative mx-auto max-w-5xl px-4 sm:px-6 lg:px-8">
<div dangerouslySetInnerHTML={{ __html: content }} />
</div>
</article>
</div>
);
};
export default PostLayout;